Python function decorator
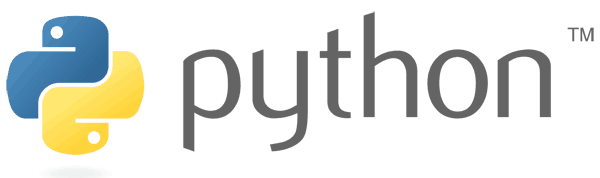
This guide will show you how to create a Python function decorator with a few easy steps, to help you separate different concerns in code. This guide does not aim to provide reference material regarding decorators, but rather explain the basics and provide a starting point for implementing your own decorator.
What is a decorator?
A Python decorator is similar to a Java annotation if you are familiar with the JVM language, where you decorate or “annotate” a function prefixing the decorator’s name with @
. For example, the decorator below publishes the function hello()
on the url /
on a Flask application:
@app.route('/') def hello() -> str: return 'Hello world from Flask!'
More broadly speaking, a decorator is a structural design pattern that lets you add behaviour to an object, by wrapping its decorated function in a new function that has the same signature.
Read More »Python function decorator